Who doesn’t love Github? It’s home to thousands of developers and open-source projects. To get a better understanding of the power and flexibility of React Hooks, we have 10 React Hooks Example examples from their excellent documentation.
1. React hooks for async communication
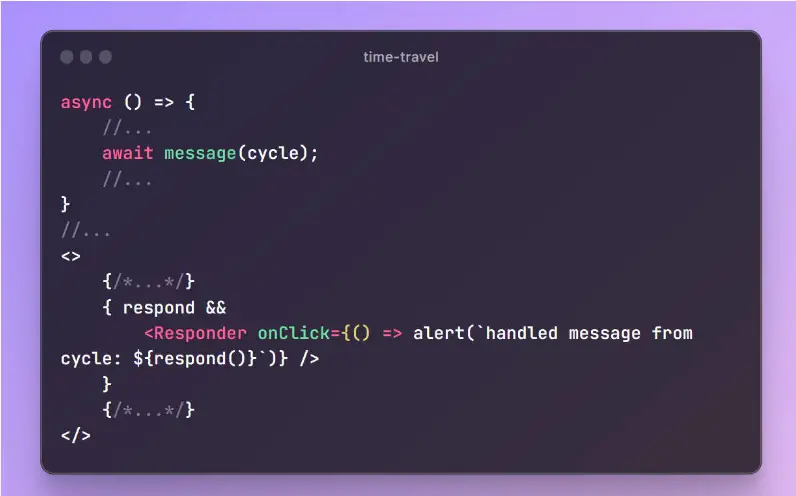
time-travel
React hooks for async communication
exports
The two most important exports of this module are:
Usage:
const [state, setState, getState] = useRefState();
This hook can be used to interact with the current state of the component from a process spawned from an old render cycle.
async () => { //... const fresh = getState(); setState(fresh.process()); //... }
useFuture
// Sends a message for a render cycle in the future to respond to export function useFuture<A, B>(): [undefined | ((f: (a: A) => B) => void), (a: A) => Promise<B>];
Usage:
const [respond, message] = useFuture();
This hook can be used to send a message
which will force a re-render and will await for a future cycle to respond
.
async () => { //... await message(cycle); //... } //... <> {/*...*/} { respond && <Responder onClick={() => alert(`handled message from cycle: ${respond()}`)} /> } {/*...*/} </>
2. React Hooks app to calculate the BMI of a person
React Hooks app to calculate the BMI of a person. It can store the data for 7 days with the help of LocalStorage.
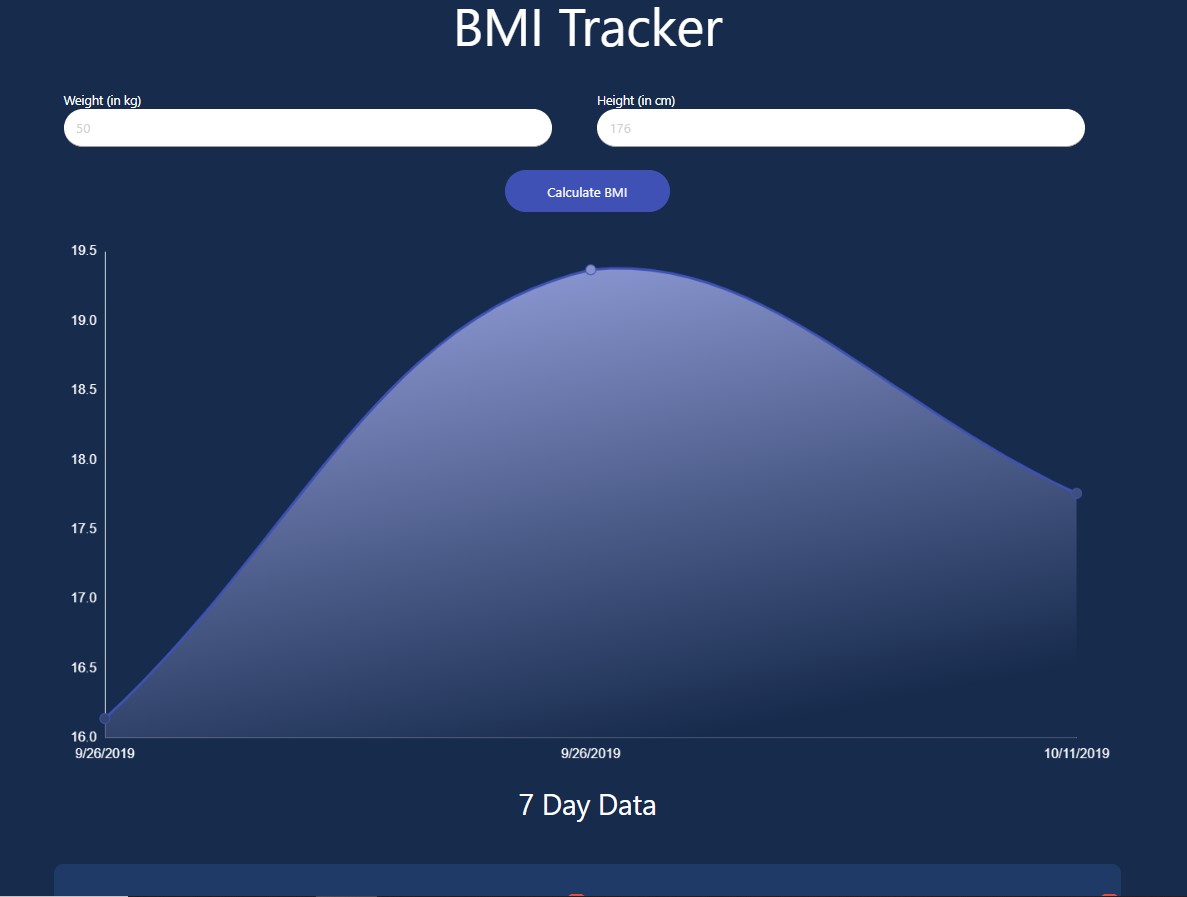
Created with create-react-app. See the full create-react-app guide.
Install
npm install
Usage
npm start
Enhancement
- Removing the dependency of the Materialize-CSS module
2. Chart going crazy on hovering over the old points
3. React hook for OpenAI Whisper with speech recorder, real-time transcription
useWhisper
React Hook for OpenAI Whisper API with speech recorder, real-time transcription and silence removal built-in
- REAL-TIME TRANSCRIPTION DEMO
- AnnouncementuseWhisper for React Native is being developed.
Repository: https://github.com/chengsokdara/use-whisper-native
Progress: chengsokdara/use-whisper-native#1
npm i @chengsokdara/use-whisper
yarn add @chengsokdara/use-whisper
4. React hook to detect component mount, unmount and re-renders
See Demo
Install
npm i use-is-rerender --save
yarn add use-is-rerender
Usage
import { useRerender } from 'use-is-rerender' const Button = () => { useRerender() return <button>Click</button> }
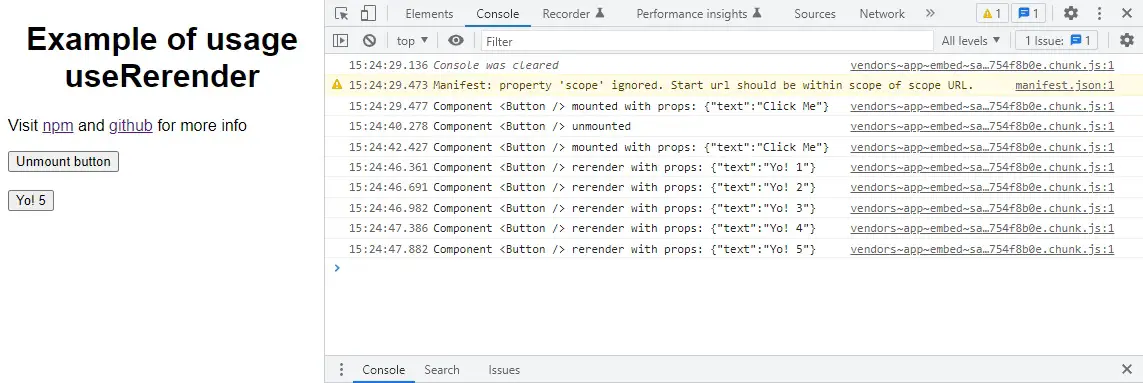
Available hook options
Option | Acceptable Values | Details |
---|---|---|
logProps | true (default)false | Can disable props logging |
Production mode
Hook not working when NODE_ENV==='production'
Read More
5. A React.js CRUD app using Redux Toolkit and RTK Query hooks
Build a CRUD App with React.js and Redux Toolkit
In this comprehensive guide, you’ll build a React.js CRUD app using Redux Toolkit and RTK Query hooks. In brief, we’ll create RTK Query hooks that React will use to perform CRUD operations against a REST API.
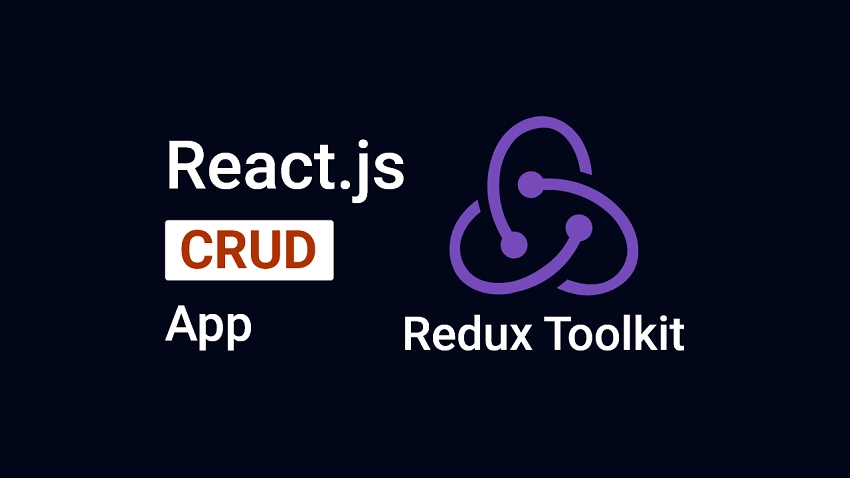
Topics Covered
- Run the React Redux Toolkit App Locally
- Run a Django API with the React App
- Setup the React Project with Tailwind CSS
- Scaffold the React Project
- Add Tailwind CSS
- Create the CRUD API Slice with RTK Query
- Add the CRUD API Slice to the Redux Store
- Create Reusable React Components
- Implement the CRUD Functionalities
- CREATE Operation
- UPDATE Operation
- DELETE Operation
- READ Operation
- Test the React CRUD App
- Perform the CREATE Functionality
- Perform the UPDATE Functionality
- Perform the READ Functionality
- Perform the DELETE Functionality
Read the entire article here: https://codevoweb.com/build-crud-app-with-reactjs-and-redux-toolkit/
6. React hook for logging per component lifecycle
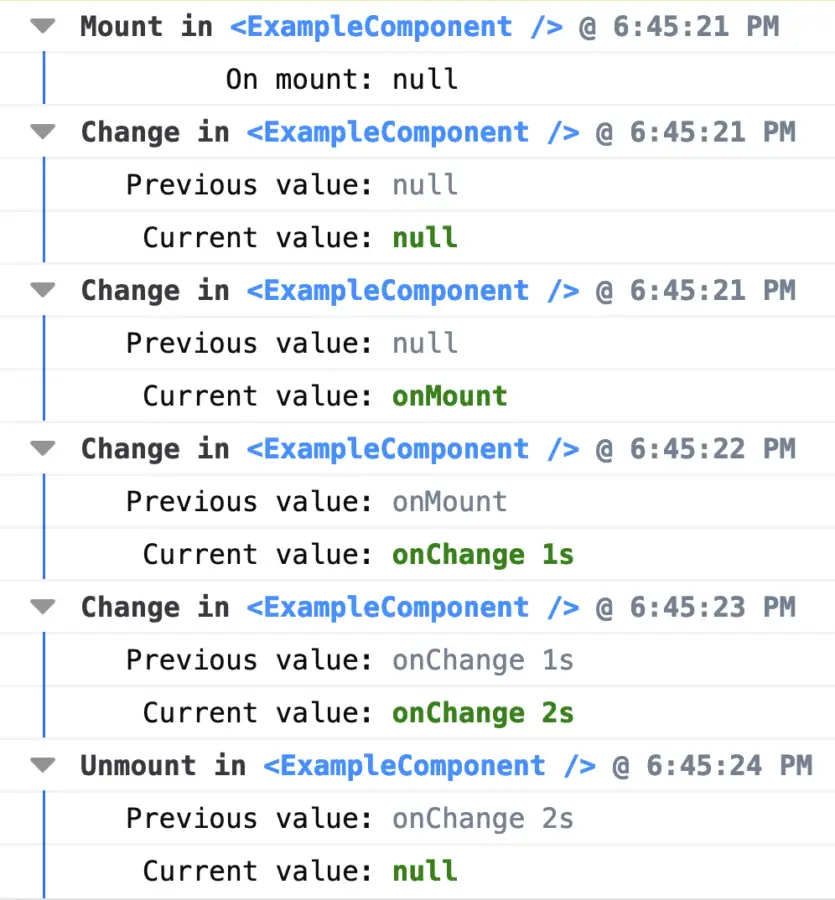
Features
- 🪶 Lightweight — under 1.5 kB gzipped & minified
- 🗂️ Typed — made with TypeScript, shipped with types
- 🥰 Simple — don’t worry about any changes in your props & state
- 🔧 Customizable — able to change everything you see in the logs
- 🔬 Tested — up to 💯% unit test coverage
- 🏎️ Fast — native react hooks & optimized
- 📭 No dependecies
7. React-persist-store
⚡ A persistent state management library for React. Create your own hooks that share data across components
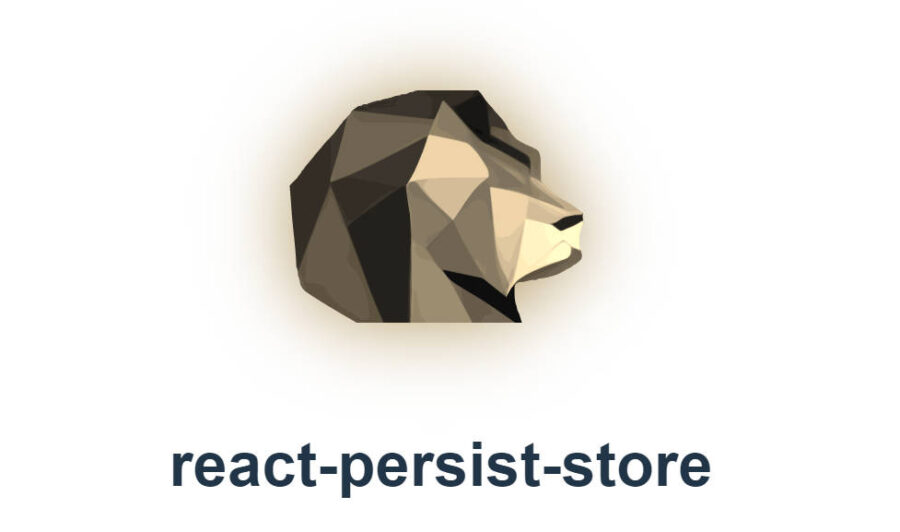
Create your own hooks that share state. Initialise your store with default values and use an API that infers type information for you.
Features
- Persistence: data is serialized and persisted to local storage by default, and hydrated automatically
- Type safety: full TypeScript integration with type inference from default values
- Reactive design: state updates are shared across components
- Simplicity: does not require any component wrapping, or use of other abstractions such as the Context API
Getting Started
At a high level, it is generally suggested to create a store.ts
file in which you set up your exported hook functions. Then these can be imported and used in components.
License
Distributed under the MIT License. See LICENSE
for more information.
8. Simple but well styled Calculator made by using hooks
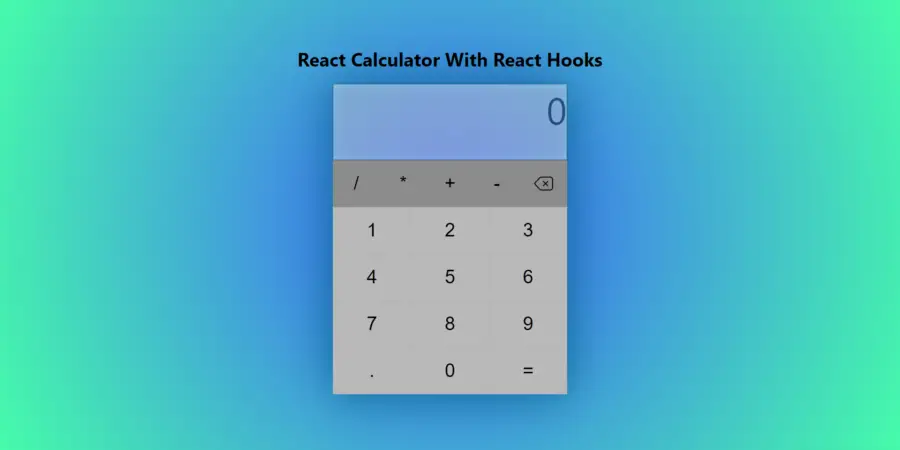
https://github.com/vasilykhromykh/React-Calculator-With-React-Hooks
MIBO UIkit
MIBO UIkit is a set of React components and hooks used to build pages on MIBO’s apps. It also contains a theme file for dark and light mode.
Install
yarn add @miboplatform/uikit
Setup
Theme
Before using MIBO UIkit, you need to provide the theme file to styled-component.
import { ThemeProvider } from 'styled-components'
import { light, dark } from '@miboplatform/uikit'
...
<ThemeProvider theme={isDark}>...</ThemeProvider>
Reset
A reset CSS is available as a globally styled component.
import { ResetCSS } from '@miboplatform/uikit'
...
<ResetCSS />
Types
This project is built with Typescript and exports all the relevant types.
9. React hook for entering a PIN code

Installation
// with npm
npm install --save react-pin-input-hook
// with yarn
yarn add react-pin-input-hook
10. Todo List App Using React and Styled Components
A TODO List React JS Project using React Hooks and Styled Components.
Local Setup
- Clone the Repository using the following command: git clone https://github.com/tndungu/TodoListApp.git
- Open the Repository using your favorite text editor. I use Visual Studio Code as a personal preference.
- Open terminal and run the following: npm install
- Run the project using npm start. This will open the project in http://localhost:3000
Video
11. Calculator made in React.JS using Hooks as useReducer
Calculadora
Descrição
- Essa é uma calculadora feita em React utilizando Hooks como
useReducer
- A sua origem vem do vídeo “The Perfect Beginner React Project” do YouTuber “Web Dev Simplified“
- O objetivo desse repositório é uma refatoração do código funcional e também a adição de testes unitários
Tecnologias
- React.JS (17)
- Vite (2.7)
GitHub Pages
- Você pode ver a aplicação em funcionamento aqui.
Imagem
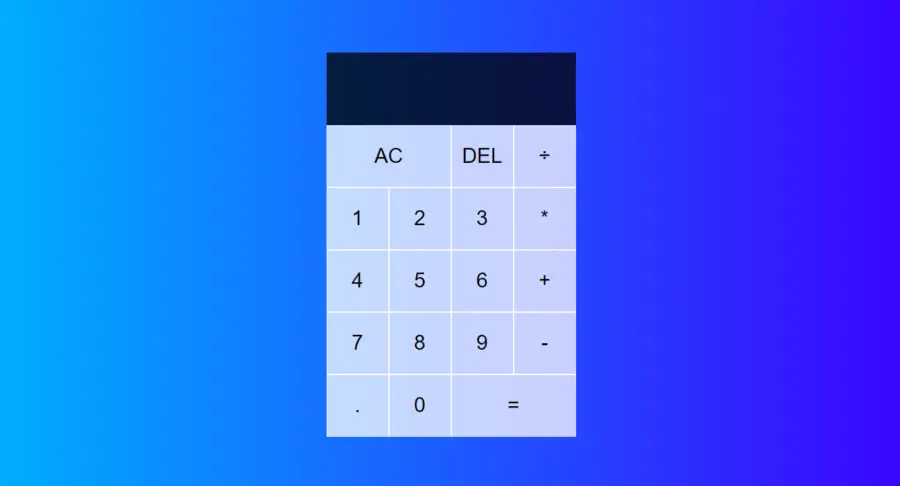
12. Nodes-navigation-focus
nodes-navigation-focus is a JS library which automates the focus moviment in the screen.
The library uses the react-hooks to manipulate the DOM and recieve and return infomation about the state of the element.
Installation
Install nodes-navigation-focus with npm
npm install nodes-navigation-focus
Features
- Manage state of DOM elements
- Move focus state of a complex layout structure
- Allows you recive informartion about the elemenet that is currently focused